Point Cloud Fetch
In this guide we establish a secure connection to a Qb2 device. Afterwards, the point cloud data is requested and one or multiple point cloud frames are received. Finally, we get data from the received point cloud and plot a single point cloud frame.
Please follow the API Guide first to install the Python package. |
Fetch point cloud from Qb2
We use the
service from PointCloud
namespace over the gRPC channel and issue a single get (retrieve one point cloud frame) or streaming request (continuously receive point cloud frames) to the device.
The code snippets below show examples of connecting to a Qb2 with hostname blickfeld_qb2.core_processing.services
and fetching point cloud frames from it.qb2-xxxxxxxxx
Get single frame
The following example shows how to fetch a single point cloud frame using the
method.Get
import blickfeld_qb2
from blickfeld_qb2.base.grpc.channel import Channel
# open secure connection to Qb2
with Channel(fqdn_or_ip="qb2-xxxxxxxxx") as channel:
service = blickfeld_qb2.core_processing.services.PointCloud(channel)
frame = service.get().frame
# print frame id
print("received frame with id", frame.id)
Continuously stream point cloud frames
The following example shows how to continuously stream point cloud frames from a Qb2 device:
import blickfeld_qb2
from blickfeld_qb2.base.grpc.channel import Channel
# open secure connection to Qb2
with Channel(fqdn_or_ip="qb2-xxxxxxxxx") as channel:
service = blickfeld_qb2.core_processing.services.PointCloud(channel)
# request point cloud stream from Qb2
for i, response in enumerate(service.stream()):
# extract point cloud frame from response
frame = response.frame
# print frame id
print("received frame with id", frame.id)
# get 3 frames and stop
if i == 2:
break
Get data from point cloud frame
In the code snippet below we read out the cartesian coordinates
for each point in the received point cloud frame.
We further use these values to calculate azimuth and elevation vectors.xyz
import numpy as np
# get x,y,z coordinates from the frame points
xyz = frame.binary.cartesian
# calculate azimuth and elevation
distance = np.sqrt(xyz[:,0] ** 2 + xyz[:,1] ** 2 + xyz[:,2] ** 2)
azimuth = np.arctan(xyz[:,0] / xyz[:,1])
elevation = np.arcsin(xyz[:,2] / distance)
Plot point cloud frame
Finally,
, azimuth
and elevation
vectors are used to plot the desired field of view from the point cloud frame as shown in the code snippet below.photon_count
import matplotlib.pyplot as plt
index = np.argwhere((azimuth > np.deg2rad(20)) & (azimuth < np.deg2rad(30)) & (elevation > np.deg2rad(5)))
plt.scatter(azimuth[index], elevation[index], c=frame.binary.photon_count[index], s=0.1)
plt.xlabel("Azimuth [rad]")
plt.ylabel("Elevation [rad]")
plt.show()
The plot result is shown in the Figure below:
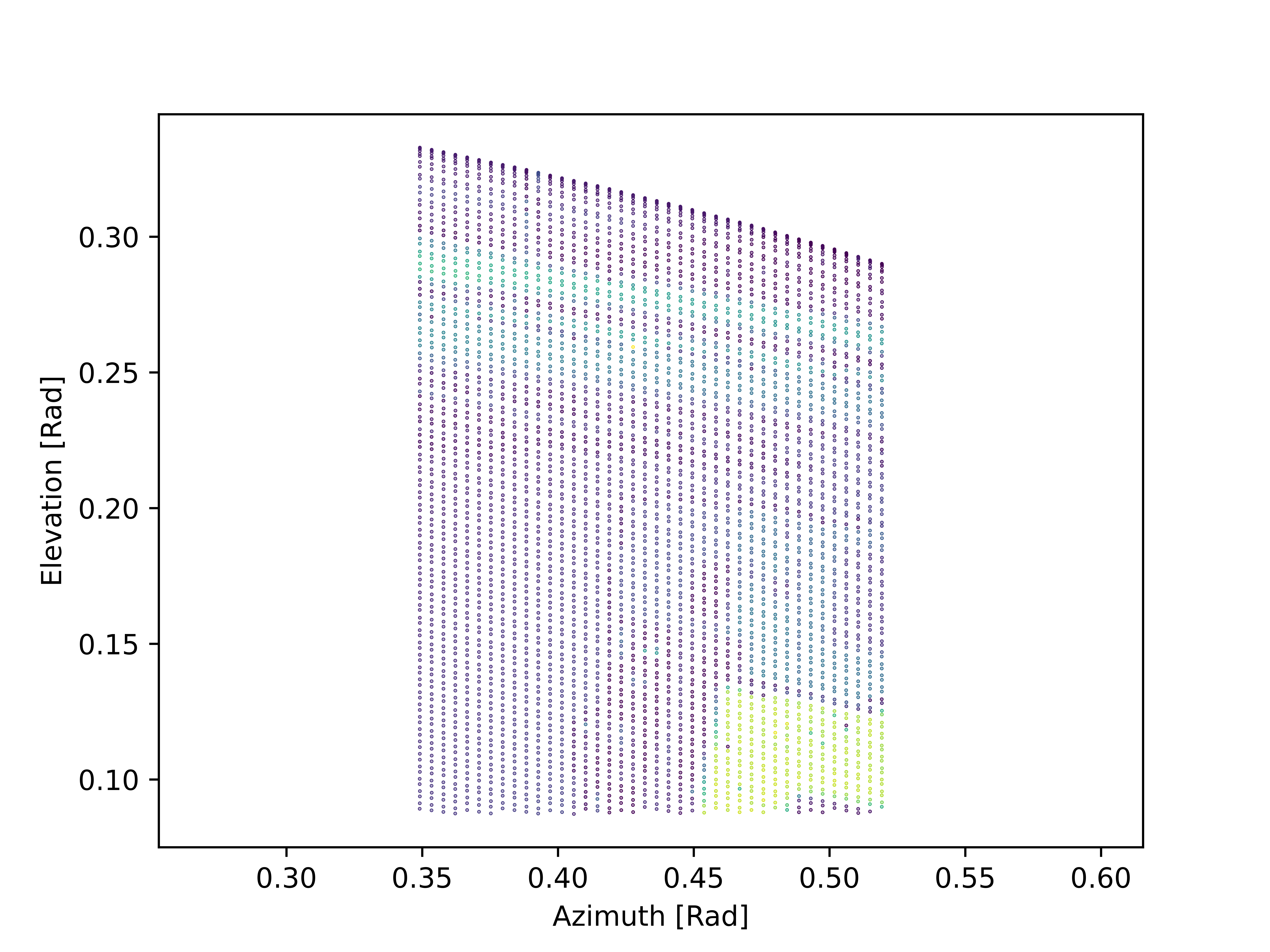